Hi all!
I'm new to VBA and code in general and I'm currently making a macro that imports point coordinates from a text file to create a spline.
I've already gleaned a lot of information online but I'm stuck on the part of creating a table from the text file.
The biggest problem I encounter is the following: splitting my values and arranging them in a table that I could call later (To place my points, compare the positions of them etc ...)
Here's where I am:
Private Sub cmdDecoupe_Click()
Dim inputString, values() As String
Dim firstValue, secondValue As String
' Chaîne de caractères
inputString = "0.0013 0.0010"
' Supprimer les espaces en trop et divise la chaîne en deux parties
values = Split(Trim(inputString), " ")
' Si la longueur de caractères est supérieur ou égale à 1 alors assigne les valeurs aux variables et remplace les points par des virgules
If UBound(values) >= 1 Then
firstValue = CDbl(Replace(values(0), ",", "."))
secondValue = CDbl(Replace(values(1), ",", "."))
' Affiche les valeurs dans la fenêtre de la console (à des fins de vérification)
MsgBox ("Première valeur : " & firstValue & " ;Deuxième valeur : " & secondValue)
Else
' Gère le cas où la chaîne ne peut pas être correctement divisée
MsgBox "La chaîne d'entrée n'est pas au format attendu.", vbExclamation
End If
>End Sub
However, in the message box that appears, I only have the first value that is displayed. I have the impression that the split only keeps the first cut part.
As I'm new to code/VBA, I take into account all the advice 
Hello, the problem comes from the InputString variable.
The split creates an array variable of dimension 6 hence the empty value for secondValue.
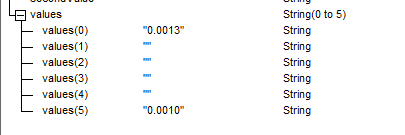
When you want to split a string, you have to master the formatting of the text file's input data with a known separator and a mastered string.
If necessary, add tests to make sure that the content of the variable is not empty and loop to get to the first one containing a data.
Another point this line seems to be useless if the data is already formatted in x.xxxx
firstValue = CDbl(Replace(values(0), ",", "."))
3 Likes
Do you really need to do it through macros?
It might be enough to import it by Curve passing through XYZ points:
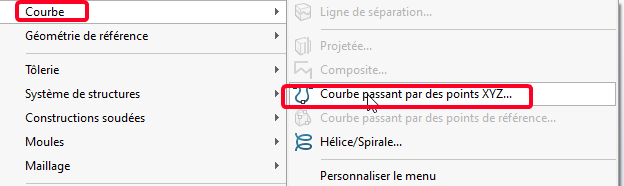
If necessary, you may just need to transform your text file to make it compatible with the function.
Otherwise I think I've already seen other macros on the subject:
or
And there are still others according to the need.
3 Likes
Hello, thank you for your answers.
@Cyril.f , I hadn't noticed that my painting was much bigger than that. I thought that the split would have kept only 2 elements, the right part of " " and the left part. If I replace with this:
values = Split(Trim(inputString), " ")
Would I only have 2 elements left in my painting?
@sbadenis , it's true that this solution is faster, the problem is that the curve I'm going to generate must have a sharp edge. If I go through this function, then the edge is rounded. Unless there is an argument for this function that allows me not to have continuity in tangency?
Regarding The CAD Coder's " Create a spline " macro. I had the opportunity to take a look at it and it works. Except that my big block is to create an array with the values. In its code, THE CAD CODER does a simple increment, where I need to retrieve the data from my text file one by one.
My text is input data that I can't change.
A small additional question more general: Where can I find a list of solidworks VBA commands? I can see the object explorer but that doesn't explain the function.
Like what:
Function CreateSpline3(PointData, Surfs, Direction, SimulateNaturalEnds As Boolean, Status) As Object
How can I know the types of data requested for each argument?
Thank you
Hello
The Trim feature removes spaces before and after text, not right in the middle of it.
I haven't seen any changes to your code, it's still the same line so the result will be the same (6-dimensional array).
Two options, either use the Mid function and remove the spaces with the Trim function or do the initial split and then delete the empty values in the array so that it is the right size.
Below is a snippet of code that allows you to transfer data from an array to a temporary array to remove blank rows and resize it:
For i =0 To UBound(values)
If values(i) <> "" Then
ReDim Preserve tmpValues(j)
tmpValues(j) = values(i)
j = j + 1
End If
Next i
ReDim values(UBound(tmpValues))
For j = 0 To UBound(tmpValues)
values(j) = tmpValues(j)
Next j
Otherwise then you have to play with the chain manipulations.
For SW API Help: CreateSpline3 Method (ISketchManager) - 2023 - SOLIDWORKS API Help
3 Likes